Managing Data
Learn how to upsert, search and delete vectors in the vector store.
Before inserting data into the vector store, a collection must be created. To create a collection, please follow the guidelines on the Managing Collections page.
Once you've created your first collection, you can easily starting managing your data.
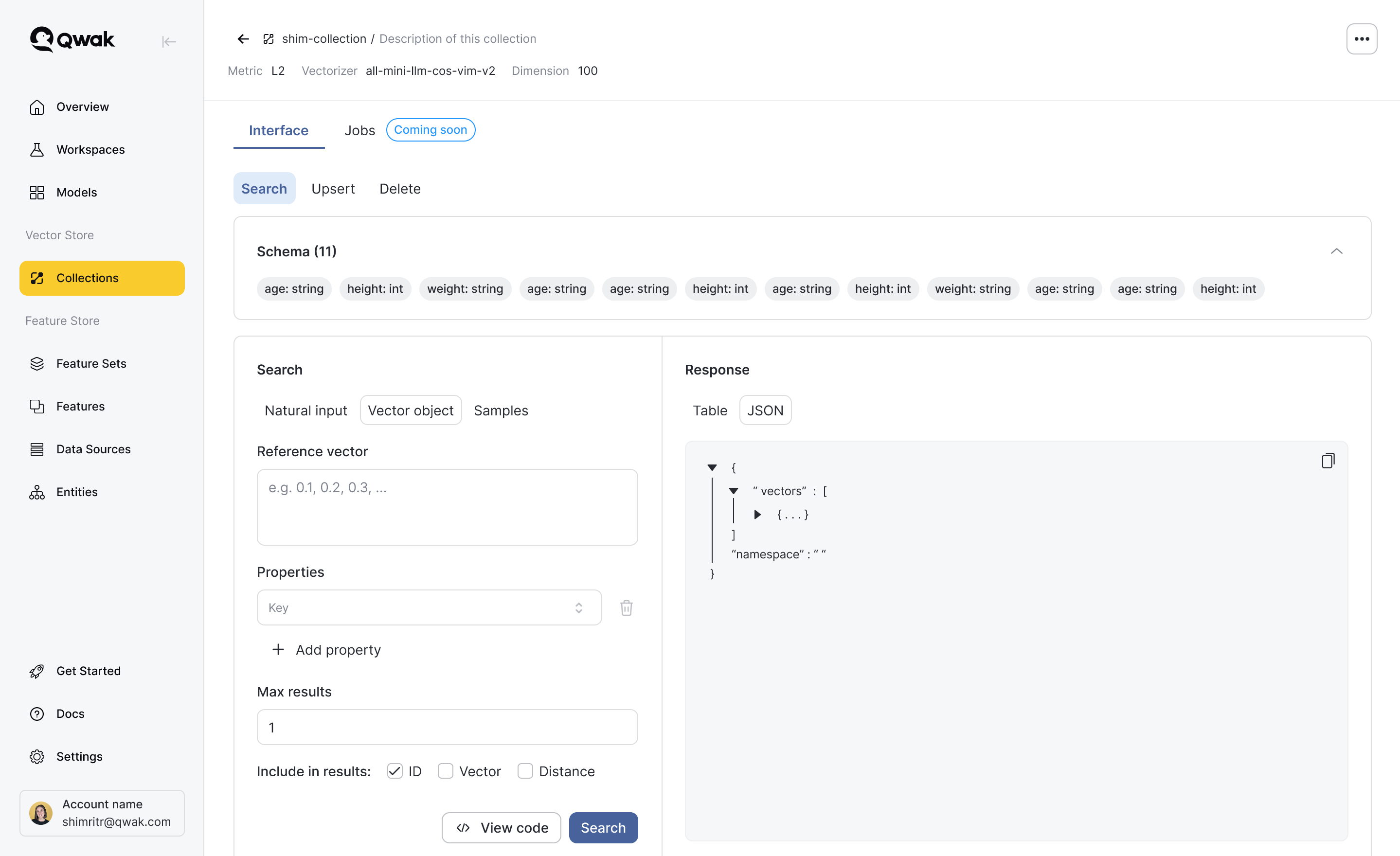
Creating a collection
For this tutorial, we'll use an example collection named
product_catalog
:from qwak.vector_store import VectorStoreClient client = VectorStoreClient() catalog_collection = client.create_collection(name="product_catalog", description="A product catalog", dimension=3, metric="cosine")
Upserting vectors
Upserting vectors allows you to either add new or replace existing vectors.
- Each vector must have a unique UUID4 formatted ID.
- The vector size must match the dimension of the collection
from qwak.vector_store import VectorStoreClient
client = VectorStoreClient()
collection = client.get_collection_by_name("product_catalog")
collection.upsert(
ids=[
"70165628-d42c-48b7-bba1-f86006b201c0"
],
vectors=[
[0.1, 0.2, 0.3]
],
properties=[{
"name": "Shoes",
"color": "yellow",
"size": "large"
}]
)
Searching vectors
Searching vectors allows your to find vector similarity based on a reference vector
- The vector size must match the dimension of the collection
- Choose properties to include in the vector response.
- The similarity distance and matched vectors may be optionally returned in the response
from qwak.vector_store import VectorStoreClient
client = VectorStoreClient()
collection = client.get_collection_by_name("product_catalog")
search_results = collection.search(output_properties=["name", "color", "size"],
vector=[0.1, 0.2, 0.3],
top_results=5,
include_id=True,
include_vector=False,
include_distance=False
)
Deleting vectors
Deleting a vector is made by supplying the UUID of the saved vector.
from qwak.vector_store import VectorStoreClient
client = VectorStoreClient()
collection = client.get_collection_by_name("product_catalog")
collection.delete(
vector_ids=["70165628-d42c-48b7-bba1-f86006b201c0"]
)
Updated 9 months ago